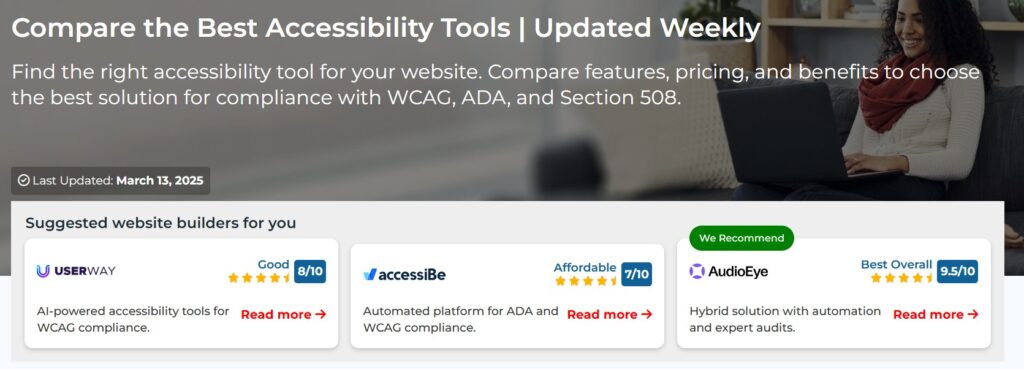
Interactive ARIA Widgets
ARIA widgets are essential for making web applications accessible to all users. When built correctly, these interactive elements work for everyone—whether they use a mouse, keyboard, or screen reader. This article breaks down practical steps for implementing accessible ARIA widgets, with real code examples and testing methods to ensure your interactive elements meet accessibility standards while staying WCAG compliant.
Understanding the Role of ARIA in Interactive Components
ARIA (Accessible Rich Internet Applications) adds semantic meaning to HTML elements that screen readers and other assistive technologies can understand. For developers, ARIA serves as a bridge between visual interactions and non-visual access methods.
When building interactive elements like modals, dropdown menus, tabs, or custom form controls, ARIA helps communicate their purpose, state, and behavior to users of assistive technologies. This is how we make sure everyone can use our web applications, regardless of how they access content.
According to the W3C’s fourth rule of ARIA use: “All interactive ARIA controls must be usable with the keyboard”. This means if you build a widget that users can click, tap, or drag, you must also make sure it works with keyboard controls.
When Native HTML Elements Aren’t Enough
Standard HTML elements like buttons, select menus, and checkboxes already have built-in accessibility features. Browsers automatically handle keyboard interactions and communicate their roles to assistive technologies. For example, a <button> element is announced as a button to screen readers and can be activated with Enter or Space keys without any extra code.
The problem? Native HTML elements have limited styling options. When you need a dropdown that looks and behaves differently from a standard <select>, you might create a custom widget. This is where many accessibility issues begin.
Custom widgets lose the built-in accessibility of native elements. You need to manually add back all those accessibility features using ARIA attributes and JavaScript. If you don’t, keyboard users and screen reader users can’t use your custom widget.
Real-world examples where you might need custom widgets with ARIA:
- Multi-level dropdown navigation menus
- Autocomplete search fields
- Custom date pickers
- Interactive charts and visualizations
- Modal dialogs with complex interactions
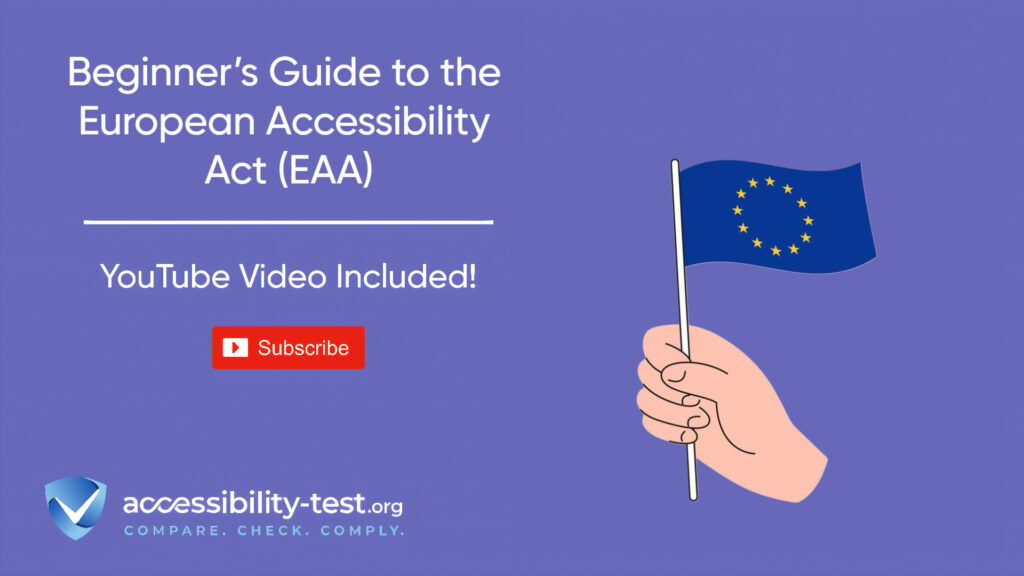
ARIA States vs. Properties | Critical Differences
ARIA includes both states and properties that serve different purposes in making interactive elements accessible.
They reflect the current condition of an element that can change during user interaction:
- aria-checked: Is this checkbox checked?
- aria-expanded: Is this dropdown menu open?
- aria-hidden: Should this element be hidden from screen readers?
- aria-selected: Is this option currently selected?
ARIA properties define fixed characteristics or relationships:
- aria-label: Provides a text label for elements without visible text
- aria-labelledby: Points to another element that serves as the label
- aria-describedby: References an element providing additional description
- aria-controls: Indicates which element is controlled by this one
The key difference? States change as users interact with your widget (handled by JavaScript), while properties generally remain static after initial setup.
Building Accessible Custom Widgets Step-by-Step
Creating accessible widgets involves several key aspects: choosing appropriate ARIA roles, managing keyboard interactions, handling focus, and updating states dynamically. Let’s look at practical implementations for common widgets.
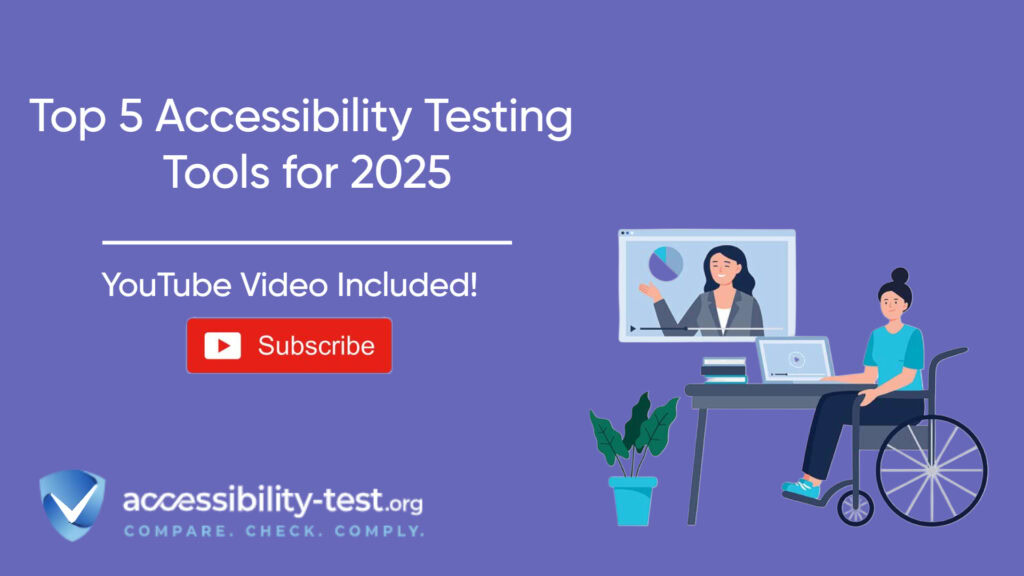
Accessible Modal Dialogs Implementation
Modal dialogs present unique accessibility challenges: they appear above other content, trap focus while open, and need to return focus when closed. Here’s how to build an accessible modal dialog:
First, structure your HTML with proper ARIA attributes:
<div>
<div>
<h2>Account Settings</h2>
<p>Update your account preferences below.</p>
<form>
<button type=”submit”>Save Changes</button>
<button type=”button” id=”cancelBtn”>Cancel</button>
</form>
<button type=”button” id=”closeBtn” aria-label=”Close”>×</button>
</div>
</div>
This HTML includes several important ARIA attributes:
- role=”dialog” tells screen readers this is a dialog box
- aria-labelledby connects the dialog to its heading
- aria-describedby links to a description of the dialog’s purpose
- aria-modal=”true” indicates this is a modal dialog that blocks interaction with page content
Next, let’s add JavaScript to handle opening and closing the modal with proper focus management:
const modal = document.getElementById(‘myModal’);
const closeBtn = document.getElementById(‘closeBtn’);
const cancelBtn = document.getElementById(‘cancelBtn’);
let lastFocus; // Stores the element that had focus before modal opened
function openModal() {
// Save the current focus position
lastFocus = document.activeElement;
// Show the modal
modal.style.display = ‘block’;
// Find the first focusable element and focus it
const firstFocusable = modal.querySelector(‘button, [href], input, select, textarea’);
if (firstFocusable) {
firstFocusable.focus();
}
// Add event listeners for closing
closeBtn.addEventListener(‘click’, closeModal);
cancelBtn.addEventListener(‘click’, closeModal);
document.addEventListener(‘keydown’, handleEscapeKey);
// Trap focus within modal
modal.addEventListener(‘keydown’, trapFocus);
}
function closeModal() {
// Hide the modal
modal.style.display = ‘none’;
// Return focus to the element that had it before
if (lastFocus) {
lastFocus.focus();
}
// Remove event listeners
document.removeEventListener(‘keydown’, handleEscapeKey);
modal.removeEventListener(‘keydown’, trapFocus);
}
function handleEscapeKey(e) {
if (e.key === ‘Escape’) {
closeModal();
}
}
function trapFocus(e) {
// Get all focusable elements
const focusableElements = modal.querySelectorAll(
‘button, [href], input, select, textarea, [tabindex]:not([tabindex=”-1″])’
);
const firstElement = focusableElements[^0];
const lastElement = focusableElements[focusableElements.length – 1];
// Handle tab navigation
if (e.key === ‘Tab’) {
if (e.shiftKey && document.activeElement === firstElement) {
// If Shift+Tab on first element, go to last element
e.preventDefault();
lastElement.focus();
} else if (!e.shiftKey && document.activeElement === lastElement) {
// If Tab on last element, go to first element
e.preventDefault();
firstElement.focus();
}
}
}
This JavaScript handles several critical accessibility functions:
- Saves the element that had focus before the modal opened
- Moves focus to the first focusable element inside the modal
- Traps keyboard focus within the modal (prevents tabbing outside)
- Allows closing with the Escape key
- Returns focus to the original element when the modal closes
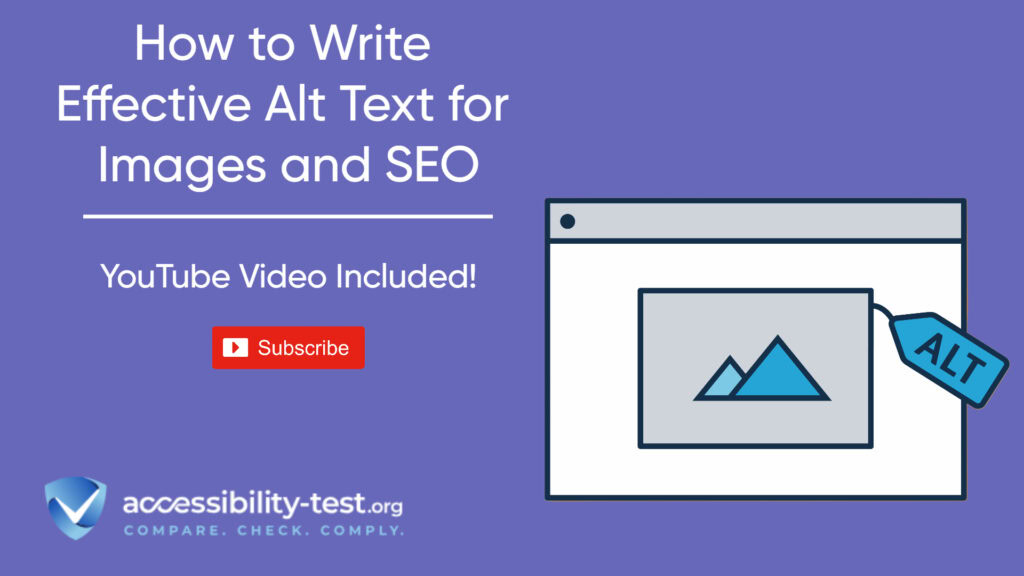
Focus Management Techniques
Focus management is essential for all interactive widgets, not just modals. Poor focus management creates confusion for keyboard users who may lose track of their position on the page.
For effective focus management:
- Always move focus to newly displayed content like modals or expanded panels
- Return focus to a logical position when content is closed or collapsed
- Maintain a predictable tab order that follows the visual layout
- Make the currently focused element visually obvious with a clear focus indicator
Focus trapping in modals prevents users from tabbing to elements that are visually hidden behind the modal. This technique creates a better experience for keyboard users who would otherwise get lost tabbing to invisible elements.
The aria-modal library provides a ready-made solution for accessible modal dialogs with these features built-in:
- Focus management
- Keyboard interaction support (Tab, Escape)
- Document scrolling management
- Backdrop click handling
- Animation support
Custom Dropdown Menus with Keyboard Navigation
Custom dropdown menus are common accessibility trouble spots. Let’s create an accessible dropdown that works with mouse, keyboard, and screen readers:
<div>
<div>
Select an option
</div>
<ul>
<li><button role=”option” aria-selected=”false” class=”btn”>Option 1</button></li>
<li><button role=”option” aria-selected=”false” class=”btn”>Option 2</button></li>
<li><button role=”option” aria-selected=”false” class=”btn”>Option 3</button></li>
</ul>
</div>
The HTML structure includes several ARIA attributes to communicate the dropdown’s purpose and state to assistive technologies:
- role=”combobox” indicates this is a combo box component
- aria-haspopup=”listbox” tells screen readers this has a popup listbox
- aria-expanded states whether the dropdown is open or closed
- role=”option” marks each item as a selectable option
- aria-selected indicates which option is selected
- aria-live=”polite” announces changes to the selected option
Now let’s add JavaScript for keyboard support:
document.addEventListener(“DOMContentLoaded”, function () {
const customSelect = document.querySelector(“.custom-select”);
const selectedOption = document.querySelector(“.selected-option”);
const optionsList = document.querySelector(“.options-list”);
const buttons = document.querySelectorAll(“.btn”);
let focusedIndex = -1;
// Toggle dropdown when clicking the select
customSelect.addEventListener(“click”, function () {
const isExpanded = optionsList.style.display === “block”;
optionsList.style.display = isExpanded ? “none” : “block”;
customSelect.setAttribute(“aria-expanded”, !isExpanded);
if (!isExpanded) {
// When opening, set focus on first option
focusedIndex = 0;
buttons[^0].focus();
}
});
// Select option when clicking a button
optionsList.addEventListener(“click”, function (event) {
if (event.target.tagName === “BUTTON”) {
selectOption(event.target);
}
});
// Keyboard support for the dropdown trigger
customSelect.addEventListener(“keydown”, function (event) {
switch (event.key) {
case “Enter”:
case ” “:
// Toggle dropdown with Enter or Space
event.preventDefault();
const isExpanded = customSelect.getAttribute(“aria-expanded”) === “true”;
optionsList.style.display = isExpanded ? “none” : “block”;
customSelect.setAttribute(“aria-expanded”, !isExpanded);
if (!isExpanded) {
focusedIndex = 0;
buttons[^0].focus();
}
break;
case “ArrowDown”:
// Open dropdown and focus first option
event.preventDefault();
optionsList.style.display = “block”;
customSelect.setAttribute(“aria-expanded”, “true”);
focusedIndex = 0;
buttons[^0].focus();
break;
case “Escape”:
// Close dropdown
optionsList.style.display = “none”;
customSelect.setAttribute(“aria-expanded”, “false”);
break;
}
});
// Keyboard support for navigating options
optionsList.addEventListener(“keydown”, function (event) {
switch (event.key) {
case “ArrowUp”:
// Navigate to previous option
event.preventDefault();
if (focusedIndex > 0) {
focusedIndex–;
buttons[focusedIndex].focus();
}
break;
case “ArrowDown”:
// Navigate to next option
event.preventDefault();
if (focusedIndex < buttons.length – 1) {
focusedIndex++;
buttons[focusedIndex].focus();
}
break;
case “Home”:
// Go to first option
event.preventDefault();
focusedIndex = 0;
buttons[^0].focus();
break;
case “End”:
// Go to last option
event.preventDefault();
focusedIndex = buttons.length – 1;
buttons[focusedIndex].focus();
break;
case “Enter”:
case ” “:
// Select current option
event.preventDefault();
selectOption(buttons[focusedIndex]);
break;
case “Escape”:
// Close dropdown and return focus to trigger
event.preventDefault();
optionsList.style.display = “none”;
customSelect.setAttribute(“aria-expanded”, “false”);
customSelect.focus();
break;
}
});
// Helper function to select an option
function selectOption(optionElement) {
// Update the visible text
selectedOption.textContent = optionElement.textContent;
// Update ARIA selected state
buttons.forEach(button => {
button.setAttribute(“aria-selected”, “false”);
});
optionElement.setAttribute(“aria-selected”, “true”);
// Close the dropdown
optionsList.style.display = “none”;
customSelect.setAttribute(“aria-expanded”, “false”);
customSelect.focus();
}
});
This JavaScript implements the expected keyboard behavior for dropdown menus:
- Arrow keys navigate between options
- Enter or Space selects the current option
- Escape closes the dropdown
- Home and End keys jump to first/last options
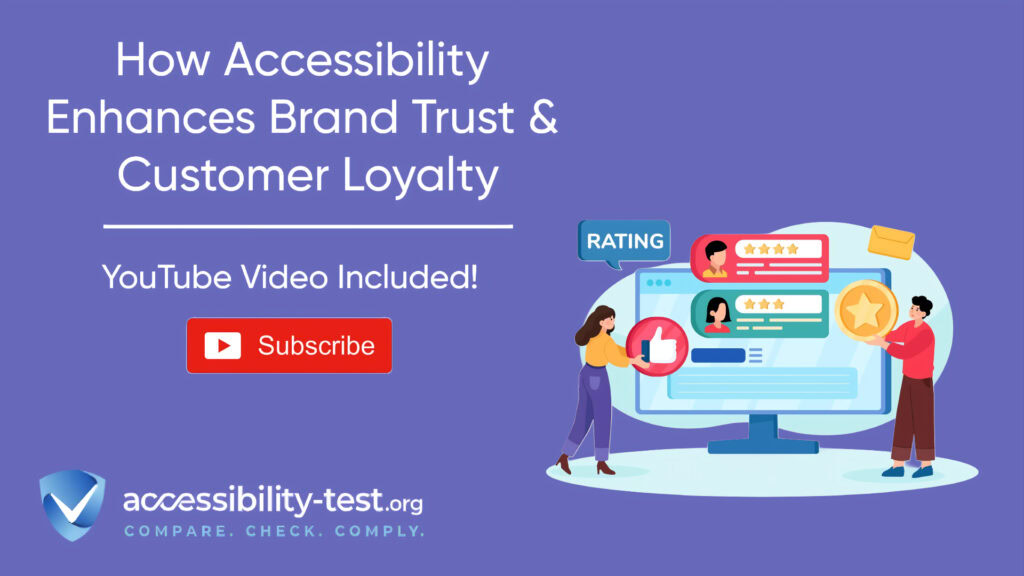
Managing ARIA Expanded States
The aria-expanded attribute plays a key role in accessible dropdowns, accordions, and other expandable widgets. It tells screen readers whether content is currently visible or hidden.
When implementing expandable widgets:
- Set aria-expanded=”false” initially (assuming the content starts collapsed)
- Update to aria-expanded=”true” when content expands
- Toggle back to aria-expanded=”false” when content collapses
This provides clear feedback to screen reader users about the current state. For example, VoiceOver might announce “popup button, collapsed” when focusing on a closed dropdown, and “popup button, expanded” when it’s open.
For accordions or disclosure widgets, apply aria-expanded to the button that controls the panel:
<button aria-expanded=”false” aria-controls=”panel1″>Section 1</button>
<div>
</div>
<script>
const button = document.querySelector(‘button’);
const panel = document.getElementById(‘panel1’);
button.addEventListener(‘click’, function() {
const isExpanded = button.getAttribute(‘aria-expanded’) === ‘true’;
button.setAttribute(‘aria-expanded’, !isExpanded);
panel.hidden = isExpanded;
});
</script>
This pattern works for any expandable widget, including navigation menus, tooltips, and tab panels.
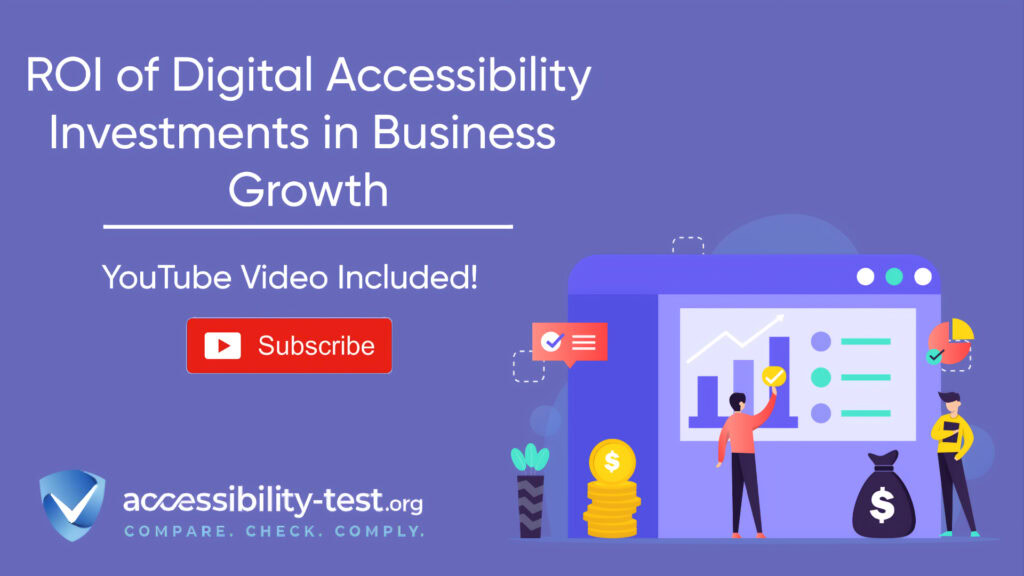
Using Automated Tools for Quick Insights (Accessibility-Test.org Scanner)
Automated testing tools provide a fast way to identify many common accessibility issues. They can quickly scan your website and point out problems that might be difficult for people with disabilities to overcome.
Visit Our Tools Comparison Page!
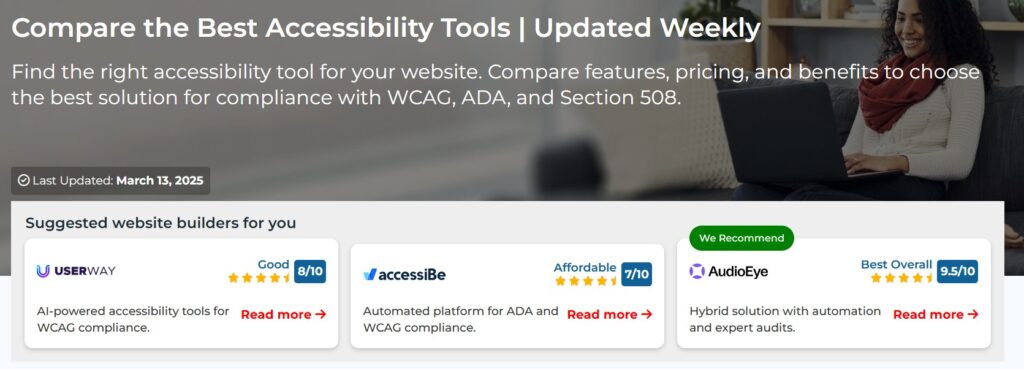
Testing Interactive ARIA Widgets
Creating accessible widgets isn’t complete until you’ve tested them with actual assistive technologies.
Screen Reader Compatibility Checklist
Testing with screen readers ensures your ARIA implementation actually works as intended. Here’s a practical screen reader testing checklist:
- Widget Identification: Does the screen reader correctly announce the widget’s role (button, dialog, listbox, etc.)?
- State Announcements: When a state changes (expanded/collapsed, selected/unselected), does the screen reader announce it?
- Instructions: Does the screen reader provide enough information for users to understand how to interact with the widget?
- Error Reporting: Are errors and validation messages properly announced?
- Dynamic Updates: When content changes dynamically, is the screen reader notified in an appropriate way?
Test with at least one screen reader on each major platform:
- NVDA (free) or JAWS on Windows
- VoiceOver on macOS
- TalkBack on Android
- VoiceOver on iOS
For screen reader testing of a modal dialog, verify that:
- The screen reader announces “dialog” when the modal opens
- The dialog title is announced
- Focus moves properly to elements inside the dialog
- You can navigate all controls within the dialog
- Escape key closes the dialog
- Focus returns to the trigger element when closed
Keyboard Navigation Test Protocol
Keyboard testing ensures people who don’t use a mouse can still operate your widgets. Follow this testing protocol for each widget:
- Access: Can you Tab to the widget from the keyboard?
- Activation: Can you activate the widget with Enter or Space?
- Navigation: Do arrow keys work as expected to navigate within the widget?
- Interaction: Can you interact with all controls using the keyboard?
- Dismissal: Can you close or exit the widget using Escape or another logical key?
- Focus Return: Does focus return to a logical place after dismissing?
For specific widgets, test these keyboard patterns:
Buttons:
- Tab to focus the button
- Press Enter or Space to activate
Dropdown Menus:
- Tab to focus the dropdown
- Press Enter, Space, or Down Arrow to open
- Use Up/Down Arrows to navigate options
- Press Enter to select an option
- Press Escape to close without selecting
Modal Dialogs:
- Tab should cycle only through elements inside the modal
- Escape should close the modal
- Focus should return to the triggering element after closing
Tab Panels:
- Tab to focus the tab list
- Use Left/Right Arrows to navigate between tabs
- Tab again to enter the active panel’s content
W3C’s “Using ARIA Keyboard Patterns” document notes: “When it comes to widgets, the ARIA keyboard pattern should be this: users can tab to the widget, then use arrow keys within the widget”. This pattern creates consistency across websites and applications.
Testing Interactive ARIA Widgets
Building accessible widgets is just the first step. Testing ensures they actually work for everyone.
Screen Reader Compatibility Checklist
Test your widgets with screen readers to verify they’re truly accessible. Here’s what to check:
- Role Announcement: Does the screen reader correctly identify the widget? For example, a tab should be announced as “tab” and a dialog as “dialog.”
- State Changes: When a widget’s state changes (like a dropdown opening), does the screen reader announce this change?
- Focus Management: Does focus move logically through the widget? Are users trapped in endless tab cycles?
- Instructions: Do users get enough information to understand how to use the widget?
Consider this testing protocol for a custom dropdown:
- Tab to the dropdown. Does the screen reader announce it’s a dropdown or combobox?
- Open the dropdown. Does the screen reader announce it’s expanded?
- Use arrow keys to navigate options. Does the screen reader announce each option?
- Select an option. Does the screen reader announce the selection?
- Close the dropdown. Does the screen reader announce it’s collapsed?
Test with at least one screen reader from each major platform (Windows, macOS, mobile) since they can behave differently.
Keyboard Navigation Test Protocol
Keyboard testing reveals whether users who can’t use a mouse can still operate your widgets effectively.
For each widget type, test specific keyboard interactions:
Buttons and Links:
- Can you Tab to the button?
- Does it show a visible focus indicator?
- Does Enter or Space activate it?
Dropdown Menus:
- Tab to the dropdown
- Press Enter or Space to open
- Use Up/Down arrows to navigate options
- Press Enter to select an option
- Press Escape to close without selecting
Modal Dialogs:
- Open the modal
- Verify focus moves inside the modal
- Tab through all interactive elements
- Try tabbing past the last element (focus should wrap to first element)
- Press Escape to close
- Verify focus returns to the element that opened the modal
Document your test results with specific issues and their impact on users. Prioritize fixes based on how severely they block users from completing tasks.
Run a FREE scan to check compliance and get recommendations to reduce risks of lawsuits
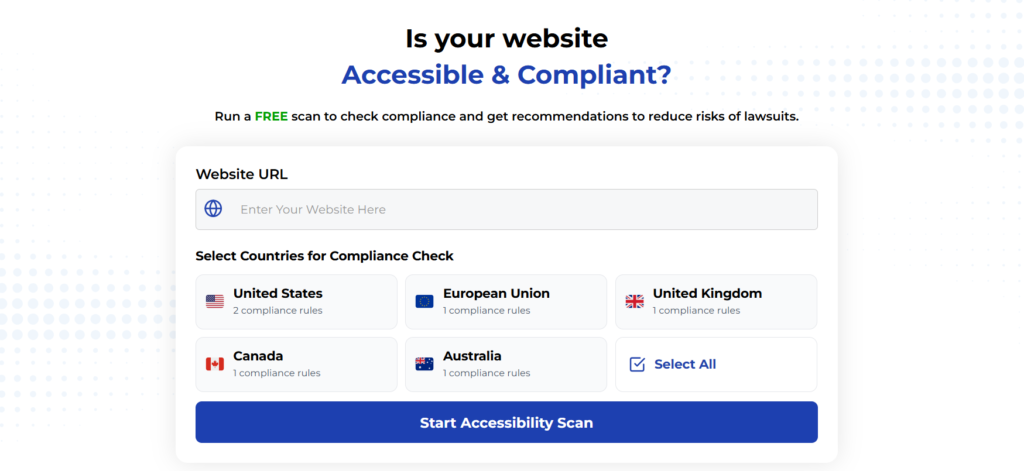
Implementing accessible ARIA widgets takes extra effort but creates a better experience for everyone. The key principles to remember:
- Only use ARIA when necessary—native HTML elements are better when they meet your needs
- Always provide keyboard support alongside ARIA attributes
- Manage focus carefully, especially when content appears or disappears
- Test with actual assistive technologies, not just automated tools
- Update ARIA states dynamically as users interact with your widgets
By following these practices, you’ll create interactive elements that work for all users, regardless of how they navigate the web.
Run a FREE scan to check compliance and get recommendations to reduce risks of lawsuits.